TL;DR - Follow Garyvee on Twitter, and you’ll probably find XSS.

Intro
When you work a desk job, sometimes you need inspiration to ‘crush it’ and Gary Vaynerchuk is my go-to hero when it comes to trying to be more productive and push forward in my career, plus he had Weshly Arms Legendary as his intro for DailyVee, which I now listen to all the time. By procrastinating more on Twitter, I saw that Gary was going to be in Planet Of The Apps, and he recommended that everyone should check out the website. When you visit the site, it looks pretty average, and it is, a very basic promotional website with a trailer embedded.
Like every normal person, whenever visiting a website, I always view the source; now by looking at the source, you could tell directly something funky is going on, check out the source if you want to figure it out on your own.
When you look at the source, you see the following iFrame:
1 | <iframe allowfullscreen='' frameborder='0' height='100%' scrolling='no' src='https://embed.apple.media/public/assets/player.html?id=59a0662a064b5400127610ba&src=https://embed.apple.media/public/embeds/59a0662a064b5400127610ba.json' |
It’s very strange to load a resource in an iFrame and then include the same origin with a JSON object through a .json resource.
From what I can grasp, embed.apple.media is used by Apple to load videos for apple music, for example the Planet of the apps trailer and a trailer for Future’s Coming Out Strong.
Investigating
Now when I first started to see if this was vulnerable to Cross-Site Scripting (XSS), I attempted to just inject the src parameter as a JavaScript URI and inject script tags to see if it would render in the HTML mark-up.
1 | https://embed.apple.media/public/assets/player.html?id=&src=javascript:alert(1) |
That didn’t work, and it’s generally not that easy, so next I looked to see what was being rendered in the .json file, and as you could see it was metadata for rendering the video.
1 | { |
The next attack scenario would be, because it wasn’t accepting arbitrary HTML or JavaScript URIs, maybe I could host my own JSON object and have see how the page renders in the HTML mark-up or evaluated by JavaScript.
So I initially used myjson.com a simple website which hosts JSON objects, and hosted the same payload that was being included on embed.apple.media, this loaded fine. The only thing which needed to be the same was the ID in the query string and the ID in the JSON object so for https://api.myjson.com/bins/139m8d.json because the ID was set as javascript:alert(1) the ID had to be https://embed.apple.media/public/assets/player.html?id=javascript:alert(1)&partner=Apple&src=https://api.myjson.com/bins/139m8d.json.
By manipulating values in the JSON object, I first included javascript URIs in values that may have been interpreted as such e.g the src, this did not work, so I started to move into and including script tags.
1 | { |
And finally, after manipulating it enough, I got my XSS in the “title” field.
So I had about 15 minutes until I was joining a friend to watch a film, so I wrote up a quick and dirty email and fired it over to product-security@apple.com
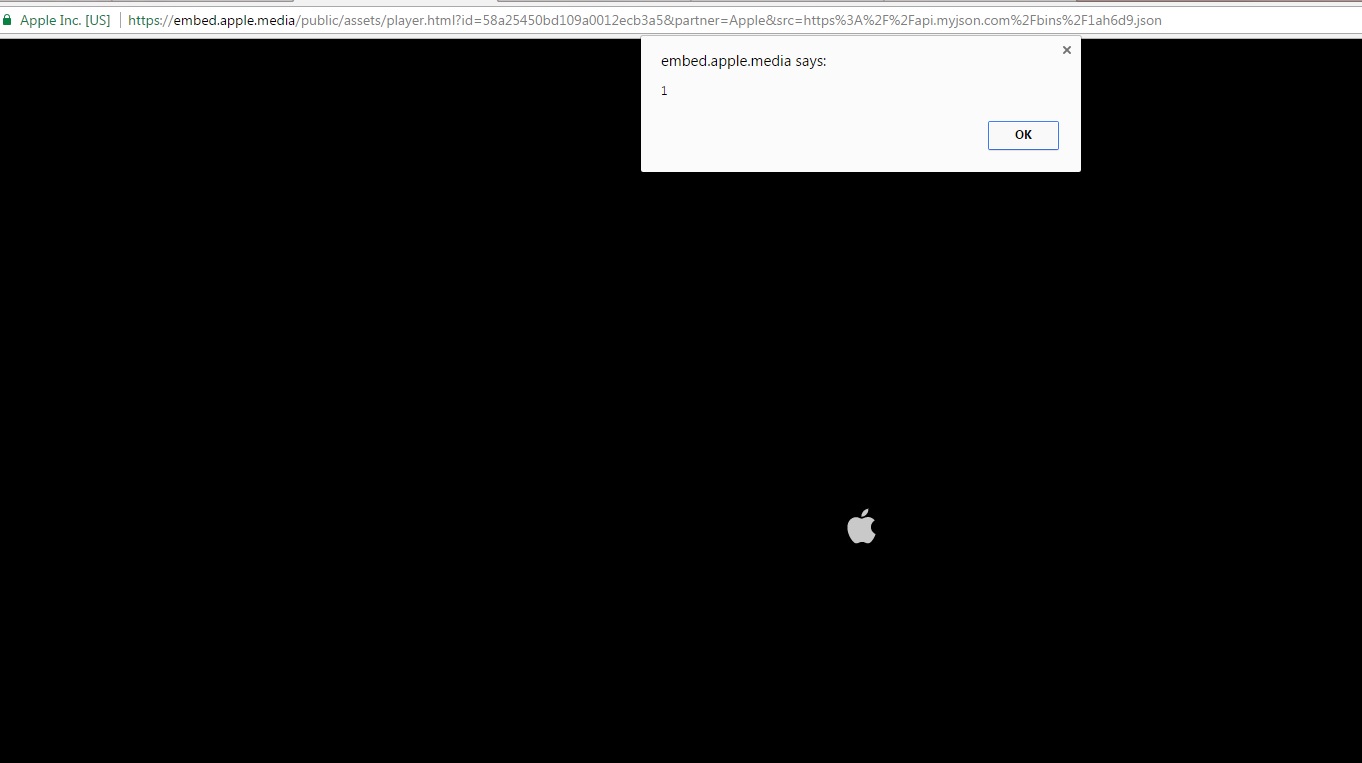
Final Tip
Something I learnt from this, h/t Alex you don’t always have to excessively load from an external resource.
You can use Data URIs to load resources in the same way.
The format, of DataURIs is data: mediatype ; base64 , data,
So in this case, you can include a Data URI of media/type application/json, with the base64 encoded data which can be interpreted as https://embed.apple.media/public/assets/player.html?id=58a25450bd109a0012ecb3a5&partner=Apple&src=data:application/json;base64,DATA.
So if you have a JSON object, you can simply base64 encode it and include it in a Data URI, and your payload becomes this, which is pretty neat.
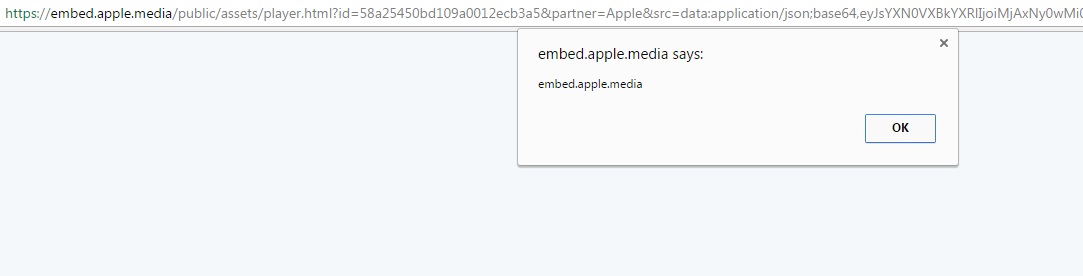
Fix
The fix appears to be that it’s only loading resources from whitelisted domains. I looked at trying to include Data URIs, and load from third-party resources over HTTP and HTTPS, and used general bypass techniques to load a third-party domain, but it appears sufficent.
The one thing that’s no-longer required is that the IDs don’t need to match, so you can just include https://embed.apple.media/public/assets/player.html?id=&src=https://embed.apple.media/public/embeds/59a0662a064b5400127610ba.json and it will still render.
Let me know if you find another way to load another resource, after you report it and they resolve it responsibly ofcourse :).
Timeline
To give Apple credit, whilst it did take a long time to resolve, embed.apple.media isn’t exactly a high priority domain, so I reported the issue in May 2017 it was resolved in January 2018, the Apple team did respond in a timely manner to all my emails.
- Initial email reporting issue May 25 2017
- Response from Apple May 26 2017
- Request on status June 12 2017
- Response from Apple June 12 2017
- Request on status October 11 2017
- Response from Apple October 12 2017
- Request on status December 12 2017
- Response from Apple December 15 2017
- Request on status February 05 2018
- Response from Apple confirming resolved February 05 2018
- Hall Of Fame 🎉